Scripting: Reading, Writing and Observing Properties
Prerequisites
First step is to open the script module, create a new script, and open the script editor. These steps are described in detail here: Writing Scripts.
To get access to the WoT functionality, include the ELCO WoT package next. Therefore, add this line at the top of the script:
const WoT = require("elco-wot");
In order to read, write and observe properties a ConsumedThing or an ExposedThing is needed. These object can be obtained as follows:
- ConsumedThing: Discovering and Consuming a Thing
- ExposedThing: Producing a Thing
For the purpose of this article, it does not matter if an exposed or a consumed thing is used. Both will be called thing in the following.
Writing a Property
To write a property, you can use the writeProperty method of an exposed or consumed thing. The following example writes the value 20.0 to a property with the title temperature:
thing.writeProperty("temperature", 20.0);
Asynchronous Execution
The writeProperty method is asynchronous and therefore returns a promise. Make sure the value has been written before continuing execution by adding the await keyword:
await thing.writeProperty("temperature", 20.0);
Reading a Property
To read a property, use the readProperty method of an exposed or consumed thing. The following example reads the property with the title temperature. Since the readProperty method is asynchronous, it returns a promise. Therefore, add the await keyword to get the actual value of the property:
let temp = await thing.readProperty("temperature")
Observing a Property
Observing a property enables the script to react to value-changes. Therefore, a callback has to be registered. The following example registers an anonymous callback to the temperature property:
thing.observeProperty("temperature", function (newValue) {
console.log("Observing current temp: " + newValue);
});
The callback has access to the new value of the property. In this example, it is just printed to the console but of course it is possible to process this data in any way fulfilling the purpose of the script.
Observer
It is only possible to register one observer. Before adding a new observer remove the old one from the property as follows:
thing.unobserveProperty("temperature");
// now it is possible to add an observer again
Asynchronous observing
The observeProperty method is asynchronous and therefore returns a promise. Making it sure the callback has been registered before resuming execution, add the await keyword:
await thing.observeProperty("temperature", function (newValue) {
console.log("Observing current temp: " + newValue);
});
Example Script
Here is an example helping to understand how reading, writing and observing properties works:
// Reading the two properties and printing them to the console
let temp = await thing.readProperty("temperature");
let temp_unit = await thing.readProperty("temperature_unit");
console.log("Current temperature is: " + temp + " " + temp_unit);
// Changing the temperature unit to °R
console.log("Writing property temperature_unit with value '°R'");
await thing.writeProperty("temperature_unit", "°R");
// Registering an observer to the property temperature_unit
console.log("==== Starting to observe the temperature_unit property ====");
await thing.observeProperty("temperature_unit", function (newValue) {
// The callback just prints the new value
console.log("Observing current temperature unit: " + newValue);
});
// Write °K as the new temperature unit
console.log("Writing property temperature_unit with value '°K'");
await thing.writeProperty("temperature_unit", "°K");
// Write °F as the new temperature unit
console.log("Writing property temperature_unit with value '°F'");
await thing.writeProperty("temperature_unit", "°F");
// Removing the observer
console.log("==== Stopping to observe the temperature_unit property ====");
await thing.unobserveProperty("temperature_unit");
// Write °C as the temperature unit again
console.log("Writing property temperature_unit with value '°C'");
await thing.writeProperty("temperature_unit", "°C");
Testing the script
Upload () and start () the script (see Process Data inside the IoTHub with Scripts for details on how to do this). The Output tab will be opened and after a while and some press at the refresh button the output should look like this:
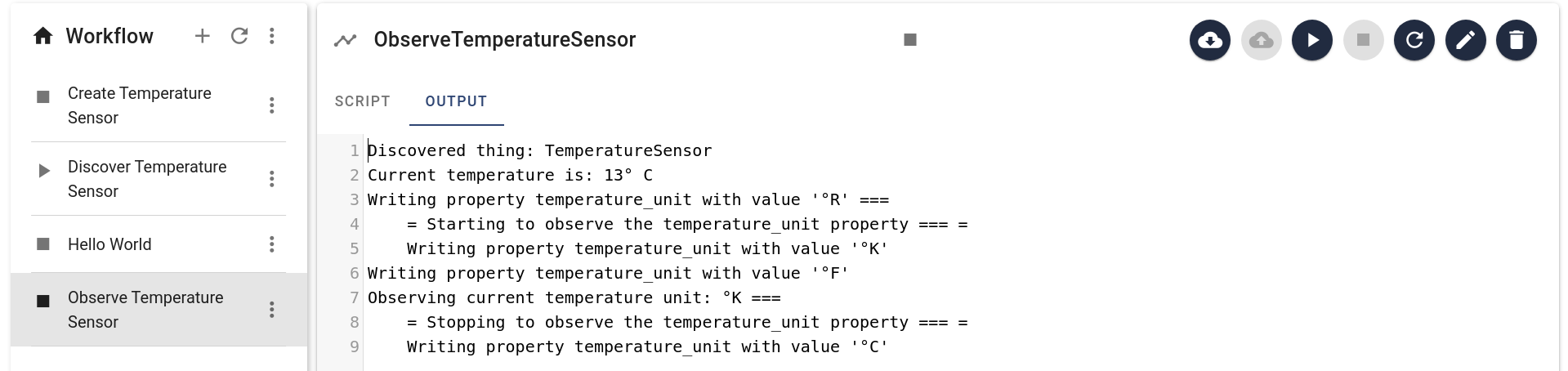
Writing and observing are asynchronous process so the sequence in the line 4,5 and 6 can be switched.
The Output of the script
This box explains the output of the script helping to understand the read, write and observe functionality. After writing the value to °R but the observer for temperature_unit is not registered yet so the new value is not printed to the console. In contrast to this, the observer gets called after writing °K and °F.
Lastly, after unregistering the observer from the temperature_unit property, it is not called again.