Scripting: Producing a Thing
Setting up the Environment
First step is to open the script module, create a new script, and open the script editor. These steps are described in detail here: Writing Scripts.
To get access to the WoT functionality, include the ELCO WoT module by adding this import instruction at the top of the script:
const WoT = require("elco-wot");
TIP
You can also check the developer documentation of the elco-wot module here.
Now the working environment is prepared and the implementation of the script can be done as described in the next steps.
Using the Thing Description Builder
In order to create a new thing, instantiate a new thing description builder first. The builder helps with configuring the thing and is created as follows:
let tdBuilder = WoT.newTDBuilder();
As a next step specify the thing description. A thing description can have the following properties:
- A title
- A name
- An optional description
- And a type. For more details about the possible types, please read Everything is a thing
Let's create an example based on the use case explained at Use Cases section.
tdBuilder.setThingDescription({
title: "TemperatureSensor",
name: "temperature_sensor",
description: "The temperature sensor in the garden." ,
type: "virtual.virtual"}
);
Now it is possible to add properties to our new thing by using the thing description builder. This is done in the code snippet below. The addProperty method takes the title of the property as an argument, in this case temperature.
The thing needs to set the type of the property too, in this case number. For all available types, please refer to: Everything is a Thing.
Moreover, the temperature is marked as non-writable by setting the readOnly attribute to true. Thus, the property cannot be written by scripts and using the UI of the IoTHub.
Additionally add a second property called temperature_unit of type string. This time, the readOnly is set to false so the property can be written.
tdBuilder.addProperty("temperature").setType("number").setAttribute("readOnly", true);
tdBuilder.addProperty("temperature_unit").setType("string").setAttribute("readOnly", false);
After adding all required properties, use the buildThingDescription method to create the thing description:
let thingDescription = tdBuilder.buildThingDescription();
The new created thing description can now be used to build a thing in the IoTHub.
Producing the Thing
The thing can be added to the IoTHub by using the thing description builder above. Call the produce method of the WoT scripting API to create the thing. Since the produce function is asynchronous, it will return a promise so use then as follows:
let exposedThing = WoT.produce(thingDescription).then(function(thing) {
console.log("Thing successfully created.");
}, function(error) {
console.log("Error during thing creation:", error);
});
Putting it all together
Following all the steps above correctly, the script should look like this:
/** Include the WoT module */
const WoT = require("elco-wot");
/** Create a ThingDescriptionBuilder */
let tdBuilder = WoT.newTDBuilder();
/** Set the basic ThingDescription */
tdBuilder.setThingDescription({
title: "TemperatureSensor",
name: "temperature_sensor",
description: "The temperature sensor in the garden." ,
type: "virtual.virtual"}
);
/** Add a non-writable property of type float64 and named temperature to the ThingDescription */
tdBuilder.addProperty("temperature").setType("number").setAttribute("readOnly", true);
/** Add a writable property of type string and named temperature_unit to the ThingDescription */
tdBuilder.addProperty("temperature_unit").setType("string").setAttribute("readOnly", false);
/** Build the ThingDescription */
let thingDescription = tdBuilder.buildThingDescription();
/** Produce the thing */
let exposedThing = WoT.produce(thingDescription).then(function(thing) {
console.log("Thing successfully created.");
}, function(error) {
console.log("Error during thing creation:", error);
});
Testing the Script
Upload () and start () the script (see Process Data inside the IoTHub with Scripts for details on how to do this). The Output tab will be opened and after a while, the message Thing successfully created should appear:
Click at the Refresh Output button () to get the latest output.

Now, the thing is added to the IoTHub. You can click on the things page ( icon in the navigation bar) and see that the thing that you just created is listed there.
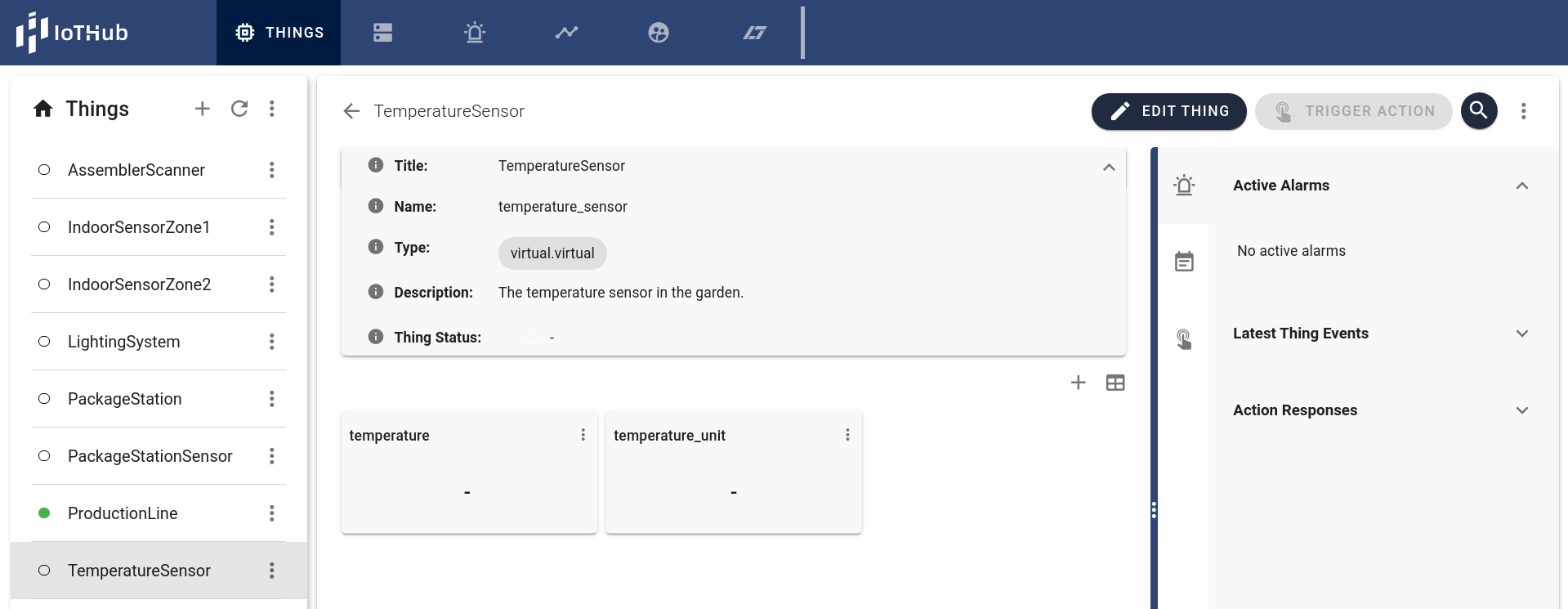